Forecasting using ARIMA (Autoregressive Integrated Moving Average) Technique.

- Published on
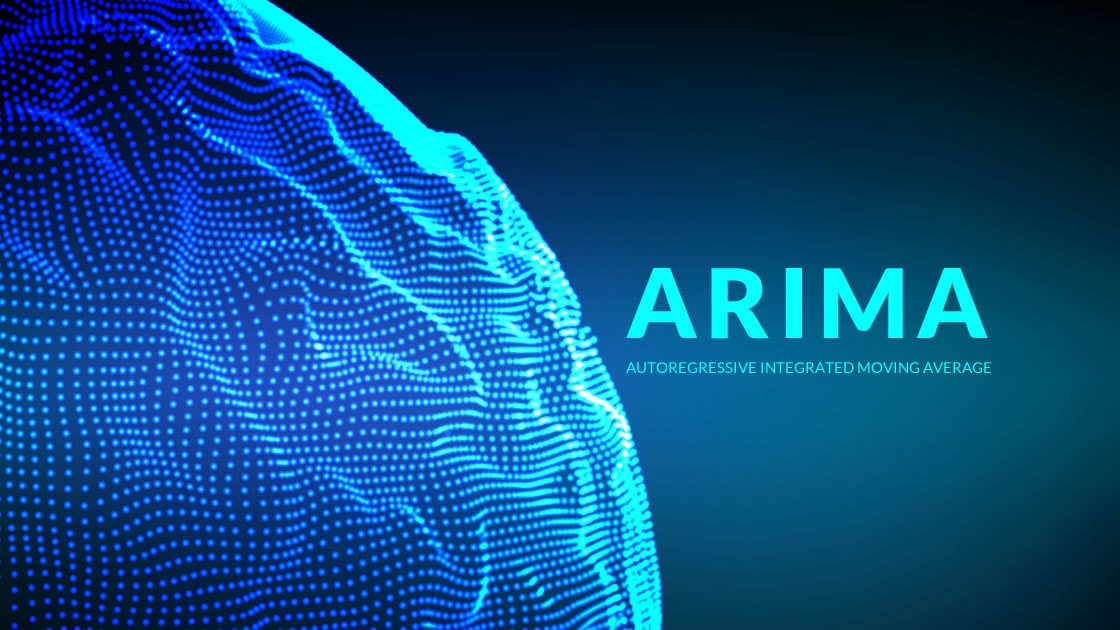
Forecasting is a crucial task in many industries, enabling businesses to make informed decisions based on future predictions. In this article, I will explore ARIMA for time series forecasting. I will provide a step-by-step guide and code examples using Python to demonstrate how to implement these techniques.
Understanding Time Series Forecasting
Before diving into the techniques, let's establish a basic understanding of time series forecasting. Time series data represents observations collected at regular intervals over time, such as daily, monthly, or yearly data points. The objective is to predict future values based on historical patterns and trends present in the data.
ARIMA (Autoregressive Integrated Moving Average)
ARIMA is a widely used technique for time series forecasting. It combines three components: autoregression (AR), differencing (I), and moving average (MA). Here are the steps to implement ARIMA:
1. Stationarity and Differencing
To apply ARIMA, the time series data must be stationary. Stationarity refers to a constant mean, variance, and autocovariance over time. We can check stationarity using statistical tests or visual inspection. If the data is not stationary, we perform differencing to make it stationary.
2. Autocorrelation and Partial Autocorrelation Analysis
Autocorrelation (ACF) and partial autocorrelation (PACF) plots help identify the order of autoregressive (AR) and moving average (MA) terms in ARIMA. These plots reveal the correlation between the time series and its lagged values.
3. Model Estimation and Forecasting
After determining the appropriate ARIMA parameters, we estimate the model using the historical data. We then validate the model by comparing predicted values with actual values. Finally, we use the model to forecast future values.
Step-by-Step Guide:
Here's a step-by-step guide on implementing ARIMA (Autoregressive Integrated Moving Average) for time series forecasting using Python:
Step 1: Import the necessary libraries
Start by importing the required libraries: pandas
for data manipulation and statsmodels
for implementing ARIMA.
import pandas as pd
from statsmodels.tsa.arima.model import ARIMA
Step 2: Load and preprocess the time series data
Load your time series data into a pandas DataFrame and preprocess it if necessary. Ensure that the data is in a suitable format with a datetime index.
# Load the time series data into a DataFrame
data = pd.read_csv('your_data_file.csv')
# Preprocess the data if necessary (e.g., convert columns to datetime)
data['Date'] = pd.to_datetime(data['Date'])
data.set_index('Date', inplace=True)
Step 3: Split the data into training and testing sets
Split the data into a training set and a testing set. The training set will be used to train the ARIMA model, while the testing set will be used to evaluate the model's performance.
train_data = data.iloc[:80] # Adjust the index based on your data
test_data = data.iloc[80:] # Adjust the index based on your data
Step 4: Determine the order of differencing (d) and lag values (p and q)
To apply ARIMA, we need to determine the appropriate values for the order of differencing (d), autoregressive (p), and moving average (q) terms. This can be done using various techniques, including visual inspection of the data and analyzing autocorrelation and partial autocorrelation plots.
# Determine the order of differencing (d)
# For example, if the data is not stationary, perform differencing until it becomes stationary
d = 1
# Determine the lag values (p and q)
# Analyze the autocorrelation (ACF) and partial autocorrelation (PACF) plots
p = 1
q = 1
Step 5: Train and fit the ARIMA model
Train the ARIMA model using the training set by fitting it to the data.
# Create and fit the ARIMA model
model = ARIMA(train_data, order=(p, d, q))
arima_model = model.fit()
Step 6: Generate predictions and evaluate the model
Use the trained ARIMA model to generate predictions on the testing set and evaluate its performance.
# Generate predictions on the testing set
predictions = arima_model.predict(start=len(train_data), end=len(train_data) + len(test_data) - 1)
# Evaluate the model's performance (e.g., calculate metrics like RMSE or MAE)
Step 7: Visualize the results
Plot the actual values from the testing set and the predicted values to visualize the model's performance.
import matplotlib.pyplot as plt
# Plot actual values and predicted values
plt.plot(test_data.index, test_data['Value'], label='Actual')
plt.plot(test_data.index, predictions, label='Predicted')
# Customize the plot
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('ARIMA Forecast')
plt.legend()
# Show the plot
plt.show()
That's it! You have successfully implemented ARIMA for time series forecasting using Python. Remember to adjust the code based on your specific dataset and requirements. Additionally, you can explore further by tuning the model parameters or incorporating other techniques like seasonal differencing (SARIMA) or model diagnostics.