Forecasting using SARIMA (Seasonal Autoregressive Integrated Moving Average) Technique

- Published on
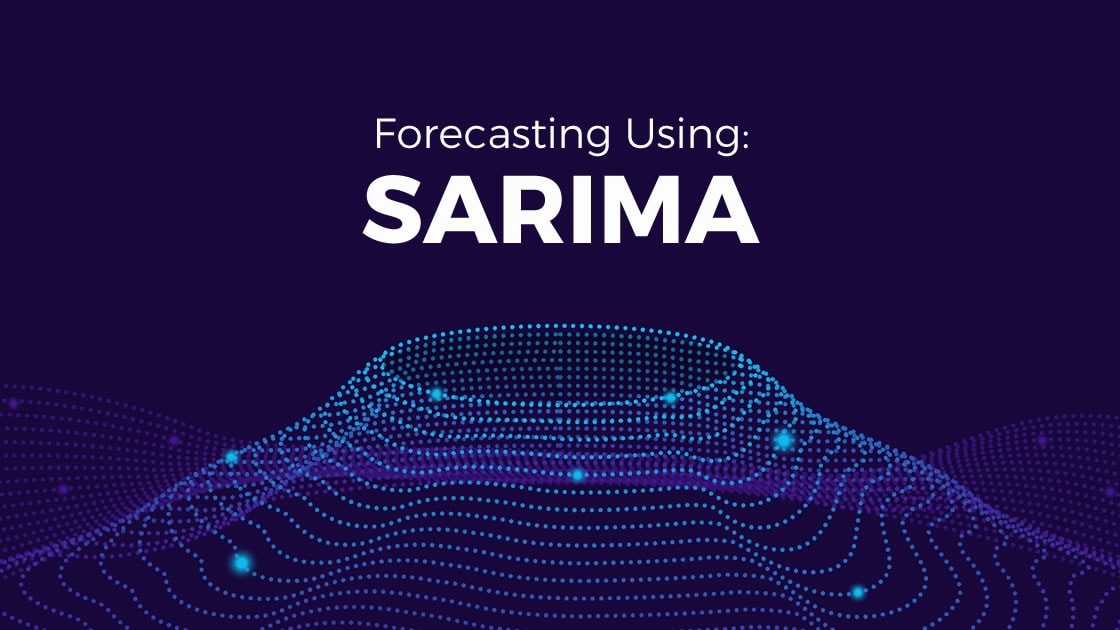
Forecasting is a crucial task in many industries, enabling businesses to make informed decisions based on future predictions. In this article, I will explore SARIMA for time series forecasting. I will provide a step-by-step guide and code examples using Python to demonstrate how to implement these techniques.
Understanding Time Series Forecasting
Before diving into the techniques, let's establish a basic understanding of time series forecasting. Time series data represents observations collected at regular intervals over time, such as daily, monthly, or yearly data points. The objective is to predict future values based on historical patterns and trends present in the data.
SARIMA (Seasonal Autoregressive Integrated Moving Average)
SARIMA extends ARIMA to handle time series data with seasonality. It incorporates additional parameters to capture seasonal patterns. Here's an overview of implementing SARIMA:
1. Incorporating Seasonality in ARIMA
We identify and analyze the seasonal patterns in the time series data. The seasonal component can be observed by plotting the data or by using methods like seasonal decomposition.
2. Seasonal Differencing and Model Estimation
Similar to ARIMA, we perform differencing to achieve stationarity. However, in SARIMA, we include seasonal differencing along with regular differencing. We estimate the SARIMA model using the differenced data and validate it.
Step-by-Step Guide:
Here's a step-by-step guide on implementing SARIMA for time series forecasting using Python:
Step 1: Import the necessary libraries
Start by importing the required libraries: pandas
for data manipulation and statsmodels
for implementing SARIMA.
import pandas as pd
from statsmodels.tsa.statespace.sarimax import SARIMAX
Step 2: Load and preprocess the time series data
Load your time series data into a pandas DataFrame and preprocess it if necessary. Ensure that the data is in a suitable format with a datetime index.
# Load the time series data into a DataFrame
data = pd.read_csv('your_data_file.csv')
# Preprocess the data if necessary (e.g., convert columns to datetime)
data['Date'] = pd.to_datetime(data['Date'])
data.set_index('Date', inplace=True)
Step 3: Split the data into training and testing sets
Split the data into a training set and a testing set. The training set will be used to train the SARIMA model, while the testing set will be used to evaluate the model's performance.
train_data = data.iloc[:80] # Adjust the index based on your data
test_data = data.iloc[80:] # Adjust the index based on your data
Step 4: Determine the order of differencing (d) and seasonal order (D), as well as the lag values (p, q, P, Q)
To apply SARIMA, we need to determine the appropriate values for the order of differencing (d), seasonal differencing (D), autoregressive (p, P), and moving average (q, Q) terms. This can be done using techniques like visual inspection of the data and analyzing autocorrelation and partial autocorrelation plots.
# Determine the order of differencing (d) and seasonal order (D)
# For example, if the data is not stationary, perform differencing until it becomes stationary
d = 1
D = 1
# Determine the lag values (p, q, P, Q)
# Analyze the autocorrelation (ACF) and partial autocorrelation (PACF) plots
p = 1
q = 1
P = 1
Q = 1
Step 5: Train and fit the SARIMA model
Train the SARIMA model using the training set by fitting it to the data.
# Create and fit the SARIMA model
model = SARIMAX(train_data, order=(p, d, q), seasonal_order=(P, D, Q, S))
sarima_model = model.fit()
Step 6: Generate predictions and evaluate the model
Use the trained SARIMA model to generate predictions on the testing set and evaluate its performance.
# Generate predictions on the testing set
predictions = sarima_model.predict(start=len(train_data), end=len(train_data) + len(test_data) - 1)
# Evaluate the model's performance (e.g., calculate metrics like RMSE or MAE)
Step 7: Visualize the results
Plot the actual values from the testing set and the predicted values to visualize the model's performance.
import matplotlib.pyplot as plt
# Plot actual values and predicted values
plt.plot(test_data.index, test_data['Value'], label='Actual')
plt.plot(test_data.index, predictions, label='Predicted')
# Customize the plot
plt.xlabel('Date')
plt.ylabel('Value')
plt.title('SARIMA Forecast')
plt.legend()
# Show the plot
plt.show()
That's it! You have successfully implemented SARIMA for time series forecasting using Python. Remember to adjust the code based on your specific dataset and requirements. Additionally, you can explore further by tuning the model parameters, incorporating exogenous variables, or conducting model diagnostics to assess the model's performance.
It's worth noting that in the code snippets provided, you'll need to replace 'your_data_file.csv'
with the actual file path or dataset you are using. Additionally, ensure that you have the necessary libraries installed, such as pandas
, statsmodels
, and matplotlib
.
Feel free to customize the code further to suit your specific needs, such as adding additional model evaluation metrics, incorporating cross-validation techniques, or experimenting with different parameter values.