Mastering Web Scraping - A Comprehensive Guide with Essential Code Examples

- Published on
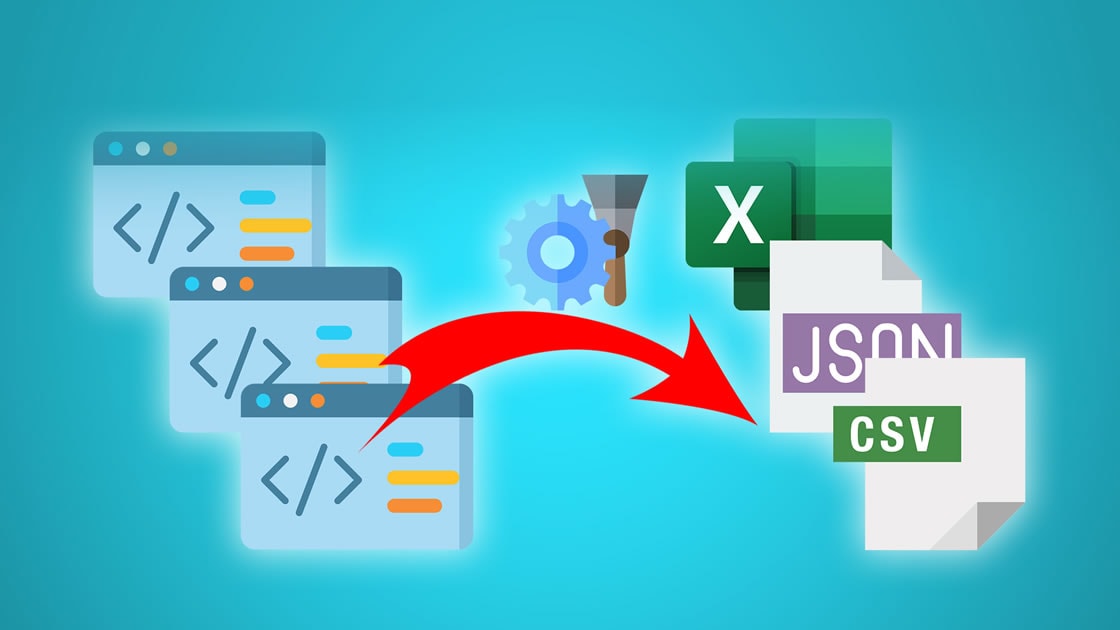
Introduction:
In the era of big data and information abundance, web scraping has emerged as a valuable technique for extracting data from websites. Whether you're a data scientist, researcher, or business professional, learning how to effectively scrape the web can provide you with a wealth of valuable information. In this article, I will explore the key concepts of web scraping and provide you with essential code examples in Python to help you get started on your web scraping journey.
Some websites can contain a very large amount of invaluable data - Stock prices, product details, sports stats, company contacts, and the list goes on. If you wanted to access this information, here’s where web scraping can help.
What is Web Scraping?
Web scraping is the process of extracting data from websites. This data can be in the form of text, images, tables, or other formats. Web scraping can be used for a variety of purposes, such as:
- Data analysis
- Research
- Price monitoring
- Lead generation
- Content curation
How Does Web Scraping Work?
Web scraping works by following these steps:
- Identify the website that you want to scrape.
- Find the data that you want to extract.
- Write code to extract the data.
- Run the code to extract the data.
The data that you want to extract can be found in a variety of places on a website. It can be in the form of text, images, tables, or other formats. The code that you write to extract the data will vary depending on the format of the data.
Web Scraping Tools
There are many web scraping tools available, both free and paid. Some of the most popular web scraping tools include:
- BeautifulSoup
- Scrapy
- Selenium
- Puppeteer
- Octoparse
These tools make it easy to extract data from websites. They provide a variety of features that make web scraping easier, such as:
- Parsing HTML
- Extracting data from tables
- Logging data
- Crawling websites
Web Scraping Process
1. Understanding the Basics of Web Scraping:
Web scraping is the process of automatically extracting data from websites by simulating human interaction with the web pages. To perform web scraping, you need to understand the following fundamental components:
a. HTML: HyperText Markup Language (HTML) is the standard markup language used to structure web pages. It provides a hierarchical structure to the content, which is essential for web scraping.
b. CSS Selectors: Cascading Style Sheets (CSS) selectors allow you to target specific elements on a web page based on their attributes. CSS selectors are invaluable in identifying and extracting data from HTML elements.
c. HTTP Requests: The Hypertext Transfer Protocol (HTTP) is used to request and deliver web pages. In web scraping, you will be making HTTP requests to retrieve the HTML content of web pages.
2. Setting Up the Environment:
Before diving into web scraping, make sure you have Python installed on your system. Additionally, you will need to install the following Python libraries:
pip install requests
pip install beautifulsoup4
3. Fetching HTML Content with Requests:
To scrape a website, start by fetching the HTML content of the target web page using the requests
library:
import requests
url = "https://www.example.com"
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
html_content = response.text
# Proceed with parsing the HTML content
else:
print("Error: Failed to retrieve the web page.")
4. Parsing HTML with BeautifulSoup: Once you have the HTML content, you can use the powerful BeautifulSoup
library to parse and extract data from the HTML structure:
from bs4 import BeautifulSoup
# Create a BeautifulSoup object
soup = BeautifulSoup(html_content, "html.parser")
# Find elements using CSS selectors
title = soup.select_one("h1").text
paragraphs = soup.select("p")
# Extract data from specific HTML attributes
link = soup.select_one("a")["href"]
image_src = soup.select_one("img")["src"]
5. Navigating the HTML Structure: Web pages often have nested HTML structures. You can navigate through the structure using various methods provided by BeautifulSoup:
# Finding parent elements
parent_div = soup.select_one("div").parent
# Finding sibling elements
next_sibling = soup.select_one("h1").find_next_sibling()
# Finding children elements
children = soup.select_one("ul").find_all("li")
Essential Code Examples
Here are some essential code examples for web scraping:
* Extracting text from a website:
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
text = soup.find("h1").text
print(text)
* Extracting images from a website:
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
images = soup.find_all("img")
for image in images:
image_url = image["src"]
image_data = requests.get(image_url).content
with open("image.jpg", "wb") as f:
f.write(image_data)
* Extracting data from a table:
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
table = soup.find("table")
rows = table.find_all("tr")
for row in rows:
cols = row.find_all("td")
data = [col.text for col in cols]
print(data)
Addendum
1. Dealing with Dynamic Content:
Some websites load data dynamically using JavaScript. To scrape such websites, you might need to use tools like Selenium WebDriver or analyze the network traffic to identify the source of the data and extract it accordingly.
2. Handling Pagination and Iterating over Multiple Pages:
Many websites display data across multiple pages. To scrape such websites comprehensively, you need to handle pagination and iterate through the pages using techniques like URL parameter manipulation or following links programmatically.
3. Respecting Website Policies and Legalities:
It is important to be a responsible web scraper and respect the website's policies. Avoid overwhelming the server with too many requests in a short period and consider implementing delay intervals between requests. Additionally, be mindful of the data you extract and how you use it, ensuring compliance with privacy laws and regulations.
4. Handling Anti-Scraping Mechanisms:
To protect their data, some websites employ anti-scraping mechanisms like CAPTCHAs or IP blocking. If you encounter such obstacles, you may need to employ techniques like using CAPTCHA-solving services or rotating IP addresses to bypass these restrictions.
5. Storing and Analyzing Scraped Data:
After successfully scraping the desired data, you can store it in various formats such as CSV, JSON, or a database. Consider using appropriate data structures and libraries to facilitate data analysis and further processing.
Conclusion:
Web scraping is a powerful tool that can be used for a variety of purposes. By understanding the basics, setting up the environment, and utilizing Python libraries like requests
and BeautifulSoup
, you can effectively scrape web pages and extract the information you need. Remember to respect website policies, handle dynamic content, and comply with legal and ethical considerations. With these tools and techniques, you'll be equipped to harness the power of web scraping for research, analysis, and decision-making purposes.
I hope that this article has provided you with a comprehensive overview of web scraping. If you have any questions, please feel free to contact me.
Note: While web scraping can provide significant benefits, it is crucial to ensure that you comply with legal and ethical guidelines. Respect website policies, terms of service, and applicable laws when engaging in web scraping activities.