Exploring the Potential of PyScript for UI Development and Web Applications

- Published on
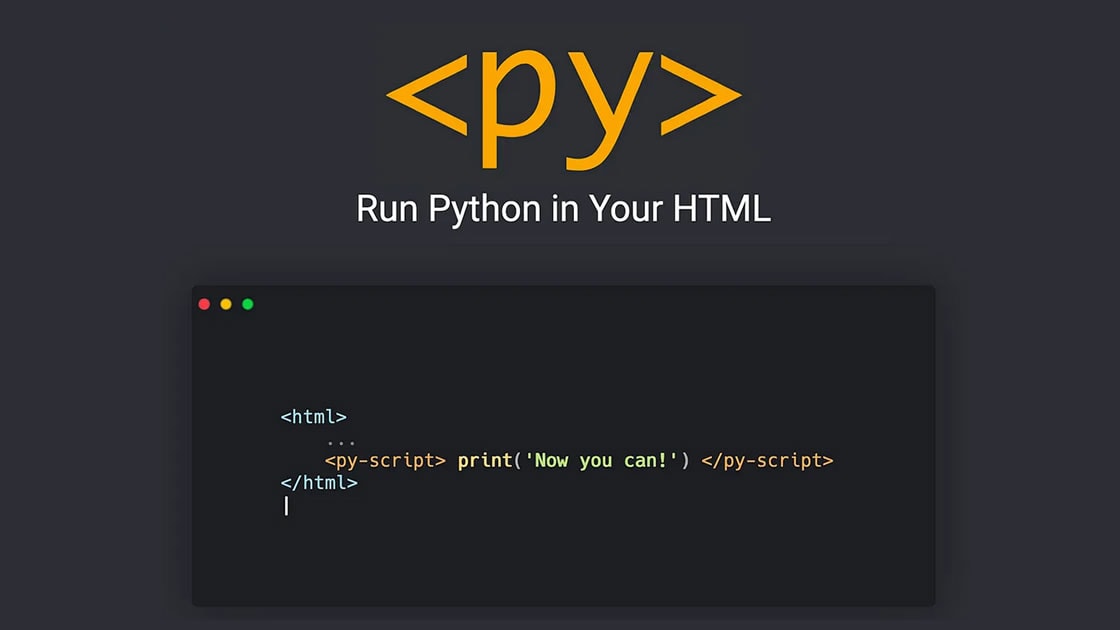
Introduction:
PyScript is a Python frontend framework that enables developers to build interactive web applications using Python for both client-side and server-side programming. With PyScript, you can leverage your existing Python skills and libraries to create dynamic web interfaces, handle user interactions, and manage data flow. This article will guide you through the process of setting up PyScript and demonstrate its usage with detailed working code examples.
Table of Contents:
- Exploring the Potential of PyScript for UI Development and Web Applications
- Why PyScript?
- Installing PyScript
- Getting Started with PyScript
- Building Interactive Web Elements
- Handling Events with PyScript
- Data Binding with PyScript
- Generating Standard Normal Distribution with NumPy and Visualizing with Matplotlib
- Some additional instances where PyScript can be beneficial are:
- Conclusion
1. Exploring the Potential of PyScript for UI Development and Web Applications
In the last couple of decades, Python and advanced UI languages like modern HTML, CSS, and JavaScript have not worked in collaboration. Python lacked a simple mechanism to create appealing UIs for simply packaging and deploying apps, while current HTML, CSS, and JavaScript can have a steep learning curve.
Allowing Python to utilize HTML, CSS, and JavaScript conventions solves not only those two problems but also those related to web application development, packaging, distribution, and deployment.
PyScript isn't meant to take the role of JavaScript in the browser, though – rather, it's meant to give Python developers, particularly data scientists, more flexibility and power.
2. Why PyScript?
PyScript offers several advantages that make it a compelling choice for Python developers looking to build web applications:
Familiarity and Reusability: PyScript allows you to leverage your existing Python skills, libraries, and knowledge. You can reuse your Python code and easily integrate popular Python libraries, making it seamless to incorporate data manipulation, scientific computations, and other functionalities into your web applications.
Pythonic Syntax: PyScript maintains the simplicity and readability of Python syntax, providing a familiar programming experience. This makes it easier for Python developers to transition into web development without needing to learn new languages or frameworks.
Full-Stack Python: With PyScript, you can use Python for both frontend and backend development. This eliminates the need to switch between different programming languages, enabling you to build end-to-end web applications entirely in Python.
Rapid Prototyping: PyScript facilitates rapid prototyping by allowing developers to quickly create interactive user interfaces using Python syntax. It simplifies the process of building and iterating on web interfaces, enabling faster development cycles.
Code Sharing: PyScript allows you to share code between the frontend and backend of your application. You can define shared functions, classes, or data structures, reducing duplication and improving code maintainability.
Integration with JavaScript: While PyScript enables you to write frontend code in Python, it seamlessly integrates with JavaScript. You can still utilize JavaScript libraries and frameworks alongside PyScript, leveraging the rich ecosystem of web development tools.
Lightweight and Easy Setup: PyScript is a lightweight framework, requiring minimal setup. It can be easily installed via pip and integrated into your existing Python development workflow.
Versatility: PyScript supports a wide range of web development use cases, from building simple interactive interfaces to more complex applications. It can be used for data visualization, web scraping, automation, educational tools, gamification, and IoT applications, among others.
Active Community and Support: PyScript benefits from an active community of developers who contribute to its growth and provide support. The framework is regularly updated, ensuring compatibility with the latest web technologies and addressing any reported issues.
Overall, PyScript combines the power of Python with web development, offering a familiar and efficient way to build interactive web applications. Its simplicity, flexibility, and integration capabilities make it an attractive choice for Python developers seeking to extend their skills to the web.
3. Installing PyScript:
To get started with PyScript, you need to install it as a Python package. Open your command prompt or terminal and run the following command:
pip install pyscript
4. Getting Started with PyScript:
PyScript provides a minimalistic and intuitive API for building web interfaces. Let's begin with a simple example:
Step 1: Create an HTML file, e.g., index.html
, and add the following content:
<!DOCTYPE html>
<html>
<head>
<title>PyScript Example</title>
<script src="https://unpkg.com/pyscript@1.0.0/dist/pyscript.js"></script>
</head>
<body>
<h1>PyScript Example</h1>
<div id="app"></div>
<script type="pyscript">
import pyscript as ps
app = ps.App("#app")
@app.route("/")
def home():
return ps.Text("Hello, PyScript!")
app.run()
</script>
</body>
</html>
Step 2: Open the HTML file in a web browser. You should see the text "Hello, PyScript!" rendered on the page.
In the above code, we import the pyscript
module and create an instance of the App
class, specifying the target element where our application will be rendered (in this case, the #app
div). We define a route for the home page ("/") and return a Text
component with the message "Hello, PyScript!".
5. Building Interactive Web Elements:
PyScript provides a set of components for building interactive web elements. Let's enhance our example by adding a button and a counter that increments on button click:
<!DOCTYPE html>
<html>
<head>
<title>PyScript Example</title>
<script src="https://unpkg.com/pyscript@1.0.0/dist/pyscript.js"></script>
</head>
<body>
<h1>PyScript Example</h1>
<div id="app"></div>
<script type="pyscript">
import pyscript as ps
app = ps.App("#app")
@app.route("/")
def home():
count = ps.State(0)
def increment():
count.value += 1
return [
ps.Text(f"Count: {count.value}"),
ps.Button("Increment", onclick=increment),
]
app.run()
</script>
</body>
</html>
In the updated code, we introduce the State
component to manage the state of our counter. We define an increment
function that increments the value of count
when the button is clicked. We render both the current count and the button in the return statement, ensuring that any changes to the state will trigger a re-render.
6. Handling Events with PyScript:
PyScript allows you to handle various events such as button clicks, form
submissions, and input changes. Let's modify our example to handle a form submission and display the submitted value:
<!DOCTYPE html>
<html>
<head>
<title>PyScript Example</title>
<script src="https://unpkg.com/pyscript@1.0.0/dist/pyscript.js"></script>
</head>
<body>
<h1>PyScript Example</h1>
<div id="app"></div>
<script type="pyscript">
import pyscript as ps
app = ps.App("#app")
@app.route("/")
def home():
name = ps.State("")
def handle_submit(event):
event.preventDefault()
ps.alert(f"Hello, {name.value}!")
return [
ps.Form(onsubmit=handle_submit, children=[
ps.Label("Name:"),
ps.Input(type="text", value=name.value, onchange=lambda event: name.set(event.target.value)),
ps.Button("Submit", type="submit")
]),
]
app.run()
</script>
</body>
</html>
In this updated code, we introduce a form element with a text input field and a submit button. The handle_submit
function is called when the form is submitted. It prevents the default form submission behavior, displays an alert with the submitted name, and clears the input field.
7. Data Binding with PyScript:
PyScript supports data binding, allowing you to synchronize the state of components with external data sources. Let's illustrate this with an example where a dropdown menu's value is bound to the selected color:
<!DOCTYPE html>
<html>
<head>
<title>PyScript Example</title>
<script src="https://unpkg.com/pyscript@1.0.0/dist/pyscript.js"></script>
</head>
<body>
<h1>PyScript Example</h1>
<div id="app"></div>
<script type="pyscript">
import pyscript as ps
app = ps.App("#app")
@app.route("/")
def home():
color = ps.State("red")
return [
ps.Select(value=color.value, onchange=lambda event: color.set(event.target.value), children=[
ps.Option("Red", value="red"),
ps.Option("Green", value="green"),
ps.Option("Blue", value="blue"),
]),
ps.Text(f"Selected color: {color.value}"),
]
app.run()
</script>
</body>
</html>
In the updated code, we use the State
component to define the color
state, which represents the selected color in the dropdown menu. The onchange
event updates the color
state based on the selected option value. The selected color is then displayed using the Text
component.
8. Generating Standard Normal Distribution with NumPy and Visualizing with Matplotlib
We will use numpy to generate numbers coming from Standard Normal distribution and then plot them using matplotlib
. We do so using the following code
<html>
<head>
<link rel="stylesheet" href="https://pyscript.net/alpha/pyscript.css" />
<script defer src="https://pyscript.net/alpha/pyscript.js"></script>
<py-env>
- numpy
- matplotlib
</py-env>
</head>
<body>
<h1>Plotting a histogram of Standard Normal distribution</h1>
<div id="plot"></div>
<py-script output="plot">
import matplotlib.pyplot as plt
import numpy as np
np.random.seed(42)
rv = np.random.standard_normal(1000)
fig, ax = plt.subplots()
ax.hist(rv, bins=30)
fig
</py-script>
</body>
</html>
This time, we also did the following:
- We defined the libraries we wanted to use in our Python environment by listing them in the
<py-env>
block, - We indicated that we will be outputting a plot by specifying it in the
<py-script>
block:<py-script output=”plot”>
.
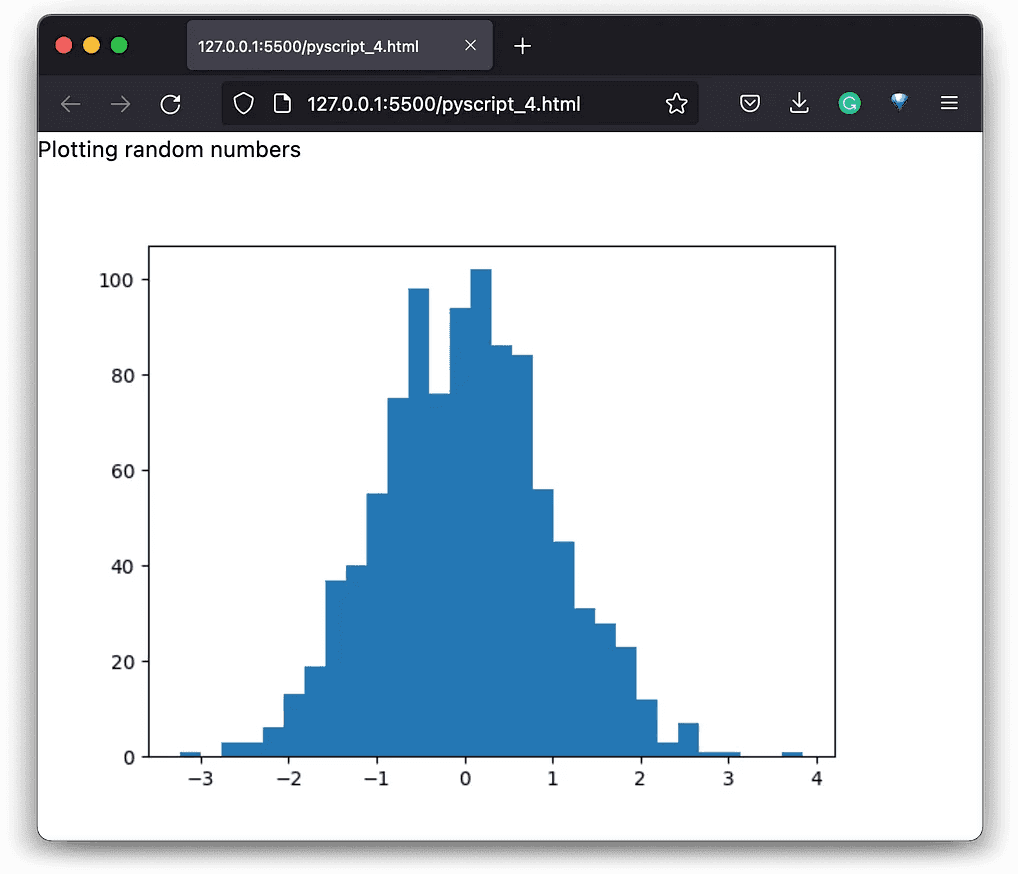
Naturally, as our codebase grows bigger, we do not have to embed it entirely in the HTML file. We can use the following block structure to load any .py script:
<py-script src="/our_script.py"> </py-script>
You can find quite a lot of examples of using PyScript here (already running in the browser) and here (code on GitHub).
9. Some additional instances where PyScript can be beneficial are:
Interactive Data Visualization: PyScript can be used to create interactive and dynamic visualizations of data. It integrates well with popular Python libraries like Matplotlib, Plotly, and Bokeh, allowing you to build interactive charts, graphs, and dashboards.
Web Scraping and Automation: PyScript can be utilized to scrape websites and automate repetitive tasks. With libraries like Beautiful Soup and Requests, you can extract data from web pages and perform actions such as form filling, clicking buttons, and navigating through webpages.
Prototyping and Proof of Concepts: PyScript enables rapid prototyping and building proof of concepts for web applications. It allows you to quickly develop interactive user interfaces using familiar Python syntax, making it easier to showcase and test ideas.
Educational Tools: PyScript can serve as a valuable tool for teaching and learning Python programming. By combining Python code and interactive web elements, educators can create engaging online tutorials, coding exercises, and interactive lessons.
Internet of Things (IoT): PyScript can be employed in IoT projects where Python is used on the frontend to interact with connected devices. It enables developers to build responsive interfaces that communicate with IoT devices, display sensor data, and control actuators.
Gamification and Interactive Experiences: PyScript can be used to create interactive games, quizzes, and other gamified experiences. By combining Python logic with HTML-based game elements, developers can build engaging and interactive web-based games.
Scientific Simulations and Modeling: PyScript can be utilized to create interactive simulations and models for scientific research and educational purposes. Python's scientific libraries, such as NumPy and SciPy, can be combined with PyScript to create interactive simulations and visualize scientific phenomena.
Rapid UI Prototyping: PyScript allows for rapid prototyping of user interfaces (UI). It enables developers to quickly iterate and experiment with different UI designs, layouts, and interactive elements.
These are just a few examples of the diverse range of applications for PyScript. With its ability to combine Python's power and simplicity with web development, PyScript offers immense flexibility in creating interactive web applications and experiences.
10. Conclusion:
PyScript offers a Pythonic approach to frontend development, enabling you to leverage your Python skills and libraries to build interactive web applications. With PyScript we will be able to run Python (and not only) code straight from our browsers. In this article, we explored the installation process for PyScript and demonstrated its usage with code examples. With PyScript, you can build web interfaces, handle events, manage state, and create dynamic user experiences using familiar Python syntax. Feel free to explore the PyScript documentation (https://github.com/pyscript/pyscript) to discover more features and capabilities offered by this Python frontend framework.