How to Create Open Graph (OG) Images for your Next.js Application

- Published on
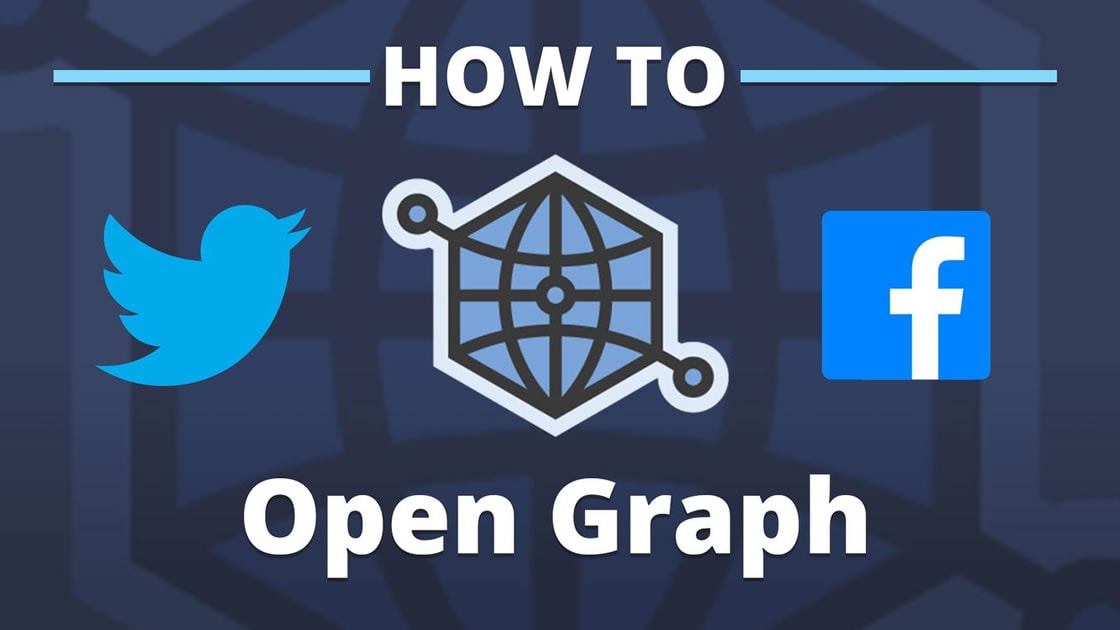
To create Open Graph (OG) images for your Next.js application, you can utilize the html2canvas
library to capture a screenshot of a specific component and generate an image file. Here's an example of how you can implement this in your Next.js application:
- Install the required dependencies by running the following command in your Next.js project directory:
npm install html2canvas
- Create a new component in your Next.js application that represents the content you want to capture as an OG image. For example, let's create a
OGImage.js
component:
import React, { useRef, useEffect } from 'react';
import html2canvas from 'html2canvas';
const OGImage = () => {
const captureRef = useRef(null);
useEffect(() => {
const captureImage = async () => {
try {
const canvas = await html2canvas(captureRef.current);
const dataURL = canvas.toDataURL('image/png');
// Here, you can save or use the generated `dataURL` as needed
console.log(dataURL);
} catch (error) {
console.error('Error capturing OG image:', error);
}
};
captureImage();
}, []);
return (
<div ref={captureRef}>
{/* Your OG image content goes here */}
<h1>Welcome to my Next.js App</h1>
<p>This is an example OG image content</p>
</div>
);
};
export default OGImage;
- Incorporate the
OGImage
component into your application where you want to generate the OG image. For instance, in your page component:
import React from 'react';
import OGImage from '../components/OGImage';
const MyPage = () => {
return (
<div>
{/* Your page content */}
<h1>Hello, Next.js!</h1>
<p>This is my awesome Next.js page.</p>
{/* OG image section */}
<div>
<h2>OG Image:</h2>
<OGImage />
</div>
</div>
);
};
export default MyPage;
- Ensure that the
MyPage
component is being rendered by a Next.js route or within a page file. When the page loads, theOGImage
component will capture its content and generate the image usinghtml2canvas
.
Remember to customize the content and styling of the OGImage
component to match your specific needs. Additionally, you can explore additional options provided by html2canvas
, such as specifying dimensions, handling transparency, or adding custom CSS.
By implementing this approach, you can dynamically generate OG images for your Next.js application based on specific components or pages. These images can then be used when sharing your application's URLs on social media platforms or other contexts where OG metadata is required.