Step-by-Step Guide on How to Build an application with the frontend in Next.js and backend in WordPress.

- Published on
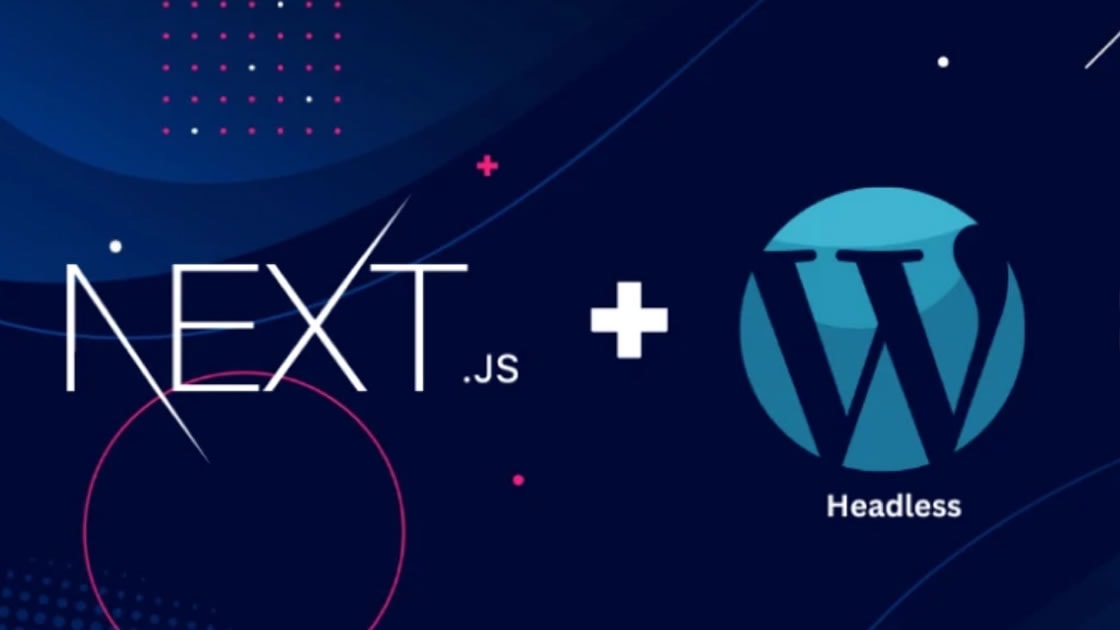
To build an application with the frontend in Next.js and the backend in WordPress, you can follow these steps:
Step 1: Set up WordPress Backend
- Install WordPress on your server or use a hosting provider that supports WordPress installation.
- Set up and configure your WordPress website following the installation instructions provided by WordPress.
- Install any necessary plugins or themes to customize your WordPress backend as per your requirements.
Step 2: Create a Next.js Project
- Install Node.js on your local machine if you haven't already. You can download it from the official Node.js website.
- Open a terminal or command prompt and navigate to the directory where you want to create your Next.js project.
- Run the following command to create a new Next.js project:
This will create a new Next.js project in a directory named "my-next-app."npx create-next-app my-next-app
Step 3: Set up API Routes in Next.js
- In your Next.js project, create a new directory called "pages/api" if it doesn't already exist.
- Inside the "api" directory, create an API route file, for example, "posts.js" to handle backend requests related to posts.
- In "posts.js," write your API route code using the Next.js API Routes syntax. For example:
export default function handler(req, res) { // Handle backend logic here, e.g., fetching posts from WordPress // Return the response as JSON res.status(200).json({ posts: [] }); }
Step 4: Fetch Data from WordPress in Next.js
- Install the "axios" package, which will be used to make HTTP requests from Next.js to WordPress:
npm install axios
- In your Next.js project, create a new directory called "lib" if it doesn't already exist.
- Inside the "lib" directory, create a file called "wordpress.js" to encapsulate the WordPress API calls.
- In "wordpress.js," write code to fetch data from WordPress using the "axios" package. For example:
import axios from 'axios'; const WORDPRESS_API_URL = 'https://your-wordpress-site/wp-json/wp/v2'; export async function getPosts() { const response = await axios.get(`${WORDPRESS_API_URL}/posts`); return response.data; }
Step 5: Use WordPress Data in Next.js Frontend
- In your Next.js project, open the page file (e.g., "pages/index.js") where you want to display the WordPress data.
- Import the
getPosts
function from "wordpress.js" and use it to fetch the posts. For example:import { getPosts } from '../lib/wordpress'; export default function HomePage({ posts }) { return ( <div> {posts.map((post) => ( <div key={post.id}>{post.title.rendered}</div> ))} </div> ); } export async function getStaticProps() { const posts = await getPosts(); return { props: { posts, }, }; }
Step 6: Start Next.js Development Server
- In your terminal or command prompt, navigate to your Next.js project directory.
- Run the following command to start the Next.js development server:
This will start the development server, and your Next.js application with WordPress integration will be accessible at http://localhost:3000.npm run dev
Now, you have a Next.js frontend application integrated with a WordPress backend. You can continue building your application by expanding the functionality based on your requirements. Here are a few additional steps you can take:
Create additional API routes in Next.js: You can create more API route files in the "pages/api" directory to handle different backend functionalities, such as creating, updating, or deleting data in WordPress. Each API route can be accessed from the frontend to interact with the WordPress backend.
Customize the frontend UI: Modify the Next.js pages and components to create your desired user interface. You can use the WordPress data fetched in the frontend to display posts, pages, categories, or any other WordPress content on your Next.js application.
Implement user authentication: If your WordPress backend has user authentication functionality, you can integrate it with your Next.js frontend. You can create login and registration pages in Next.js and use WordPress authentication APIs or plugins to handle user authentication.
Add forms and interactive features: Create forms or interactive elements in your Next.js frontend to allow users to submit data to the WordPress backend. You can use Next.js form libraries or build custom form components to handle form submissions and communicate with the WordPress APIs.
Deploy the application: Once you have completed development, you can deploy your Next.js frontend and WordPress backend separately or together. For the Next.js frontend, you can use services like Vercel, Netlify, or AWS Amplify. For the WordPress backend, you can deploy it on a hosting provider that supports WordPress deployments or use managed WordPress hosting services.
Remember to ensure proper security measures, such as validating and sanitizing user input, securing API endpoints, and implementing appropriate access controls, to protect your application and data.
These steps should give you a starting point for building an application with a Next.js frontend and a WordPress backend. You can further explore the Next.js and WordPress documentation for more advanced features and customization options based on your specific project requirements.