Top 10 WordPress Optimization Tricks & Tips for 2023

- Published on
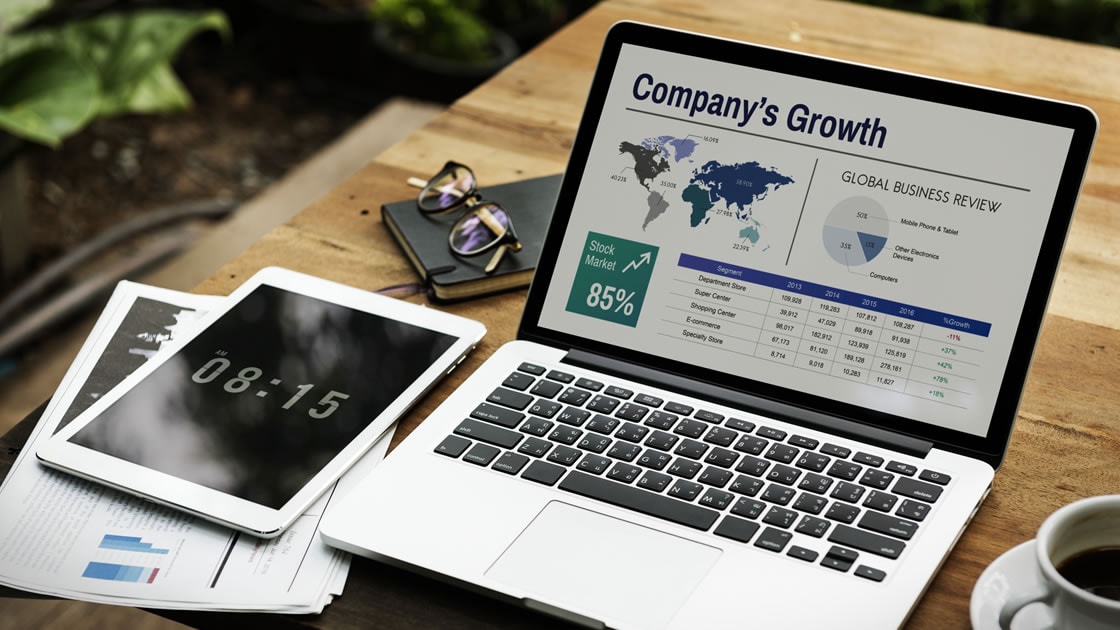
Introduction:
WordPress is the most popular content management system (CMS) in the world, powering millions of websites. To ensure optimal performance and user experience, it's crucial to optimize your WordPress site. In this article, I will explore the top 10 optimization tricks and tips for WordPress in 2023, along with practical code examples.
1. Use a Lightweight Theme:
Choosing a lightweight and well-coded theme is essential for WordPress optimization. Themes with excessive features and complex code can slow down your site. Consider using lightweight themes like Astra or GeneratePress, which offer speed and flexibility.
2. Implement Caching:
Caching significantly improves website speed by storing static versions of your pages. The most popular caching plugin for WordPress is WP Super Cache. Install and activate the plugin, and it will automatically generate static HTML files, reducing server load and improving response times.
You can implement caching using custom code, you can use the following example:
// Enable page caching
function custom_enable_page_caching() {
define('WP_CACHE', true);
}
add_action('init', 'custom_enable_page_caching');
In the code above, we're defining the WP_CACHE
constant to true
, which activates page caching in WordPress.
Place the code in your theme's functions.php
file or within a custom plugin. Ensure that the code is added before the init
action is fired.
Please note that the above code example assumes you have an appropriate caching mechanism in place at the server level. It's recommended to use caching plugins like WP Super Cache or W3 Total Cache, as they handle caching at various levels and provide more advanced features such as object caching and browser caching.
Additionally, remember to test your caching implementation thoroughly to ensure it's working as expected and doesn't interfere with any dynamic functionality or content updates on your site.
Caching plays a crucial role in improving website performance, reducing server load, and enhancing user experience by delivering cached content faster.
3. Minify and Combine CSS/JS Files:
Minifying CSS and JavaScript files reduces their file size by removing unnecessary characters and spaces. Combining multiple CSS or JS files into one reduces the number of requests made to the server. The Autoptimize plugin simplifies this process by automatically minifying and combining your site's CSS and JavaScript files.
You can achieve this functionality using custom code, you can use the following example:
// Combine and minify CSS files
function custom_combine_minify_css() {
wp_enqueue_style('custom-styles', get_template_directory_uri() . '/css/custom-styles.css', array(), '1.0.0');
wp_enqueue_style('combined-styles', get_template_directory_uri() . '/css/combined-styles.css', array('custom-styles'), '1.0.0');
}
add_action('wp_enqueue_scripts', 'custom_combine_minify_css');
// Combine and minify JavaScript files
function custom_combine_minify_js() {
wp_enqueue_script('custom-scripts', get_template_directory_uri() . '/js/custom-scripts.js', array(), '1.0.0', true);
wp_enqueue_script('combined-scripts', get_template_directory_uri() . '/js/combined-scripts.js', array('custom-scripts'), '1.0.0', true);
}
add_action('wp_enqueue_scripts', 'custom_combine_minify_js');
In the example above, we're using the wp_enqueue_style()
function to enqueue the CSS files and the wp_enqueue_script()
function to enqueue the JavaScript files. By specifying dependencies, we ensure that files are loaded in the correct order.
Place the code in your theme's functions.php
file or within a custom plugin. Modify the file paths and dependencies to match your specific CSS and JavaScript files.
Remember, manual minification and combination of CSS and JavaScript files can be a tedious process. It's generally recommended to use a reputable plugin like Autoptimize, as it handles these tasks automatically and includes additional optimizations.
4. Optimize Images:
Large image sizes can significantly slow down your site. Use image optimization plugins like Smush or EWWW Image Optimizer to automatically compress and resize images without compromising quality. Additionally, consider using modern image formats like WebP, which offer better compression.
5. Enable GZIP Compression:
GZIP compression reduces the size of files sent from your server to the visitor's browser. By enabling GZIP compression, you can significantly decrease the time it takes to transfer data. Add the following code to your .htaccess file to enable GZIP compression:
<IfModule mod_deflate.c>
# Compress HTML, CSS, JavaScript, Text, XML and fonts
AddOutputFilterByType DEFLATE application/javascript
AddOutputFilterByType DEFLATE application/rss+xml
AddOutputFilterByType DEFLATE application/vnd.ms-fontobject
AddOutputFilterByType DEFLATE application/x-font
AddOutputFilterByType DEFLATE application/x-font-opentype
AddOutputFilterByType DEFLATE application/x-font-otf
AddOutputFilterByType DEFLATE application/x-font-truetype
AddOutputFilterByType DEFLATE application/x-font-ttf
AddOutputFilterByType DEFLATE application/x-javascript
AddOutputFilterByType DEFLATE application/xhtml+xml
AddOutputFilterByType DEFLATE application/xml
AddOutputFilterByType DEFLATE font/opentype
AddOutputFilterByType DEFLATE font/otf
AddOutputFilterByType DEFLATE font/ttf
AddOutputFilterByType DEFLATE image/svg+xml
AddOutputFilterByType DEFLATE image/x-icon
AddOutputFilterByType DEFLATE text/css
AddOutputFilterByType DEFLATE text/html
AddOutputFilterByType DEFLATE text/javascript
AddOutputFilterByType DEFLATE text/plain
AddOutputFilterByType DEFLATE text/xml
# Remove browser bugs for older browsers
BrowserMatch ^Mozilla/4 gzip-only-text/html
BrowserMatch ^Mozilla/4\.0[678] no-gzip
BrowserMatch \bMSIE !no-gzip !gzip-only-text/html
Header append Vary User-Agent
</IfModule>
6. Utilize a Content Delivery Network (CDN):
A CDN stores your website's static content on servers distributed worldwide, reducing the distance between users and your site's files. This improves load times and provides a better user experience. Popular CDN services like Cloudflare and StackPath offer WordPress integration plugins that make setup a breeze.
7. Optimize your WordPress Database:
Over time, your WordPress database can become cluttered with unnecessary data, which can slow down your site. Use a plugin like WP-Optimize or WP-Sweep to clean up your database by removing spam comments, post revisions, and unused data.
Here are some practical code examples to optimize your WordPress database:
i. Using WP-Optimize Plugin: The WP-Optimize plugin provides an easy way to optimize your database. You can use the following code to run the optimization process programmatically:
// Optimize WordPress database using WP-Optimize plugin
if (class_exists('WP_Optimize')) {
$wp_optimize = WP_Optimize::get_instance();
$wp_optimize->optimize();
}
ii. Using WPDB Class: WordPress provides the wpdb
class, which allows you to execute custom SQL queries to optimize your database. Here's an example:
global $wpdb;
$tables = $wpdb->get_results("SHOW TABLES");
foreach ($tables as $table) {
$table_name = $table->{'Tables_in_' . DB_NAME};
$wpdb->query("OPTIMIZE TABLE " . $table_name);
}
iii. Using WP CLI: If you have WP CLI installed on your server, you can optimize your database using the command line interface. Run the following command:
wp db optimize
iv. Manual Optimization: If you prefer a manual approach, you can use the OPTIMIZE TABLE
SQL command for each table in your WordPress database. Here's an example of optimizing the wp_posts
table:
global $wpdb;
$wpdb->query("OPTIMIZE TABLE {$wpdb->prefix}posts");
You can repeat this query for other tables such as wp_comments
, wp_options
, and wp_postmeta
.
Please note that it's always recommended to create a backup of your database before performing any optimization tasks. Additionally, make sure you understand the implications of optimizing your database and test thoroughly to ensure your site continues to function as expected after optimization.
Remember to remove or comment out the optimization code once you have executed it to avoid running unnecessary optimization processes repeatedly.
Optimizing your WordPress database helps improve performance by reducing the overhead and enhancing query execution.
8. Enable Lazy Loading:
Lazy loading delays the loading of images and other media files until they are visible on the user's screen. This technique improves initial page load times, especially for long pages with multiple images. Install a lazy loading plugin like Lazy Load by WP Rocket or a theme that supports lazy loading out of the box.
You can achieve lazy loading with coding in WordPress. Lazy loading allows you to delay the loading of certain elements, such as images or videos, until they are visible in the user's viewport. This can significantly improve the initial page load time, especially for long pages with multiple media files.
Here's a practical code example to implement lazy loading using JavaScript:
// Add 'lazy' class to images
jQuery(document).ready(function($) {
$('img').addClass('lazy');
});
In the example above, we're using jQuery to add the lazy
class to all <img>
tags on the page. The lazy
class indicates that the images should be lazily loaded.
Next, you need to include a JavaScript library that handles the lazy loading functionality. One popular library for lazy loading is lazysizes
. You can include it by adding the following code to your theme's functions.php
file or within a custom plugin:
// Enqueue lazysizes library
function custom_enqueue_lazysizes() {
wp_enqueue_script('lazysizes', 'https://cdnjs.cloudflare.com/ajax/libs/lazysizes/5.3.0/lazysizes.min.js', array(), '5.3.0', true);
}
add_action('wp_enqueue_scripts', 'custom_enqueue_lazysizes');
This code enqueues the lazysizes
library from a CDN. Adjust the URL if you prefer to host the library locally.
Once you've added the above code, you need to modify your image tags to include the necessary attributes for lazy loading. Replace your existing image tags with the following format:
<img data-src="path/to/your/image.jpg" class="lazy" alt="Image description">
In the example above, we've replaced the src
attribute with data-src
and added the lazy
class to the <img>
tag. This informs the lazysizes library to load the image only when it becomes visible in the viewport.
Remember to update the data-src
attribute value with the actual path to your image.
By implementing lazy loading, you can optimize the loading of images and other media files on your WordPress site, resulting in faster initial page load times and improved performance.
Note: As the web evolves, native browser support for lazy loading is increasing. You can also explore using the loading
attribute with values like "lazy"
or "auto"
on your <img>
tags, but be aware of browser compatibility for the specific attribute value you choose.
9. Enable Browser Caching:
Browser caching allows returning visitors to load your site faster by storing static resources in their browser cache. By specifying expiration dates for certain types of files, you can instruct the browser to retrieve them from the cache instead of the server. Add the following code to your .htaccess file:
<IfModule mod_expires.c>
ExpiresActive On
ExpiresByType image/jpg "access plus 1 year"
ExpiresByType image/jpeg "access plus 1 year"
ExpiresByType image/gif "access plus 1 year"
ExpiresByType image/png "access plus 1 year"
ExpiresByType text/css "access plus 1 month"
ExpiresByType application/pdf "access plus 1 month"
ExpiresByType text/x-javascript "access plus 1 month"
ExpiresByType application/x-shockwave-flash "access plus 1 month"
ExpiresByType image/x-icon "access plus 1 year"
ExpiresDefault "access plus 2 days"
</IfModule>
10. Disable Unused WordPress Features:
WordPress comes with many built-in features that you may not use. Disable unnecessary features to reduce the strain on your server and streamline your site's performance. You can add the following code to your theme's functions.php file to disable features like the WordPress REST API, emojis, and XML-RPC:
// Disable REST API
add_filter('rest_authentication_errors', function ($result) {
return is_user_logged_in() ? $result : new WP_Error('rest_disabled', 'REST API is disabled.', array('status' => 403));
});
// Disable Emojis
function disable_emojis() {
remove_action('wp_head', 'print_emoji_detection_script', 7);
remove_action('admin_print_scripts', 'print_emoji_detection_script');
remove_action('wp_print_styles', 'print_emoji_styles');
remove_action('admin_print_styles', 'print_emoji_styles');
remove_filter('the_content_feed', 'wp_staticize_emoji');
remove_filter('comment_text_rss', 'wp_staticize_emoji');
remove_filter('wp_mail', 'wp_staticize_emoji_for_email');
add_filter('tiny_mce_plugins', 'disable_emojis_tinymce');
}
add_action('init', 'disable_emojis');
function disable_emojis_tinymce($plugins) {
if (is_array($plugins)) {
return array_diff($plugins, array('wpemoji'));
} else {
return array();
}
}
// Disable XML-RPC
add_filter('xmlrpc_enabled', '__return_false');
Conclusion:
Optimizing your WordPress site is crucial for improving speed, performance, and user experience. By implementing these top 10 optimization tricks and tips for WordPress in 2023, you can ensure that your site is optimized for maximum efficiency. From using a lightweight theme to implementing caching, optimizing images, enabling GZIP compression, and leveraging a CDN, each tip plays a vital role in enhancing your WordPress site's performance.
Remember to regularly optimize your WordPress database, enable lazy loading, and leverage browser caching to further boost speed and efficiency. Additionally, disabling unused WordPress features helps reduce server load and streamlines your site's performance.
By following these optimization techniques and using the provided code examples, you'll be well-equipped to create a fast and optimized WordPress website in 2023. Prioritize the user experience by delivering a seamless browsing experience that keeps visitors engaged and satisfied.
Optimization is an ongoing process, so continue to monitor your site's performance, make necessary adjustments, and stay up to date with the latest optimization practices to ensure your WordPress site remains fast, efficient, and competitive in the ever-evolving online landscape.
Happy optimizing!